Turtle graphics are a way of drawing where you control a cursor, known as the “turtle”, by instructing it how to move. For example, you tell the turtle to move forward over a certain distance, drawing a line on your screen in the process, then turn left over some number of degrees, then move forward again, and so on. If turtle graphics are well known, then that’s probably because of the the Logo programming language, in which it was an important feature. With this article, I mostly want to inform you that this elegant and fun way of creating vector graphics is available in Python too, through the turtle
module.
As an example of what it can do, let’s have a look at one obvious application of turtle graphics, which is drawing fractal curves such as the Koch snowflake (Figure 1). The Koch snowflake is made up of three segments of the Koch curve, which I have already used as an illustration in the article Fractal Dimension.
If you want to draw these kinds of curves in the classical way, then you need to do relatively convoluted computations to determine the coordinates of all the corners of the curve, to be able to draw line segments between them. Using turtle graphics, drawing them is much easier. You simply take a basic line segment and replace it with the first stage of the curve. Because the actions of the turtle are relative to its current position and orientation, you can then recursively do this again and again, down to the maximum level of detail that you want to show.
The first four stages of the Koch snowflage are shown in Figure 1. Note that the arrow-shaped turtle is visible in its final position in the illustration, after completing the fourth stage of the curve.
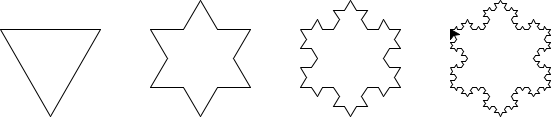
Python Code
A complete Python program that generates Figure 1 is shown below. This is a really fun program to run for yourself, since it actually creates an animation of the drawing of the shapes on your screen, which is much more interesting to look at than the final image. Try it!
from __future__ import division import turtle def segment(length, depth): if depth == 0: turtle.forward(length) else: segment(length / 3, depth - 1) turtle.left(60) segment(length / 3, depth - 1) turtle.right(120) segment(length / 3, depth - 1) turtle.left(60) segment(length / 3, depth - 1) def snowflake(size, depth): segment(size, depth) turtle.right(120) segment(size, depth) turtle.right(120) segment(size, depth) def move(distance): turtle.setheading(0) turtle.penup() turtle.forward(distance) turtle.pendown() move(-275) snowflake(100, 0) move(150) snowflake(100, 1) move(150) snowflake(100, 2) move(150) snowflake(100, 3) turtle.done()
Add new comment